Staggered animations
Warning: These pages are from before the launch of Framer X22 and are about the older animation API. These animations might still work in the current version of Framer but are officially deprecated. Learn about the current animation API here.
Meaning: a group of animations running in delayed succession (which may or may not look staggering).
Something like this:
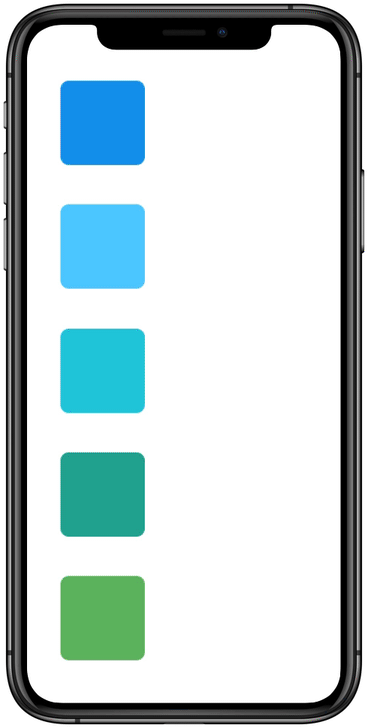
Here we also use delays, just not for all the animations. Whenever an animation needs to wait, you put a dummy animation with an await
in front of it.
First, the setup. We have 5 blocks in this example, and I will animate their horizontal position, their ‘x’ position.
const data = Data({
xPosBlockA: Animatable(40),
xPosBlockB: Animatable(40),
xPosBlockC: Animatable(40),
xPosBlockD: Animatable(40),
xPosBlockE: Animatable(40)
});
There’s an override for each of them, which binds their left
property to the respective Animatables.
export const BlockA: Override = props => {
return {
left: data.xPosBlockA
};
};
export const BlockB: Override = props => {
return {
left: data.xPosBlockB
};
};
…
Just as when delaying an animation, we use dummy animations (that don’t change anything on the screen), together with async
/ await
/ finished
for inserting breaks. (See Chaining animations for more about this.)
export const StaggeredAnimation: Override = () => {
return {
async onTap() {
// First animation – starts right away
animate(data.xPosBlockA, 234);
// Second animation – after a 0.2 second delay
await animate.ease(Animatable(0), 1, { duration: 0.2 }).finished;
animate(data.xPosBlockB, 234);
// Third animation – after another 0.2 second delay
await animate.ease(Animatable(0), 1, { duration: 0.2 }).finished;
animate(data.xPosBlockC, 234);
// Fourth animation – after yet another 0.2 second delay
await animate.ease(Animatable(0), 1, { duration: 0.2 }).finished;
animate(data.xPosBlockD, 234);
// Fifth animation – after one more 0.2 second delay
await animate.ease(Animatable(0), 1, { duration: 0.2 }).finished;
animate(data.xPosBlockE, 234);
}
};
};
download this project
By the way, I applied this StaggeredAnimation
override to the device frame, so that you can tap anywhere on the screen to start the sequence.